Mean chart code as follow
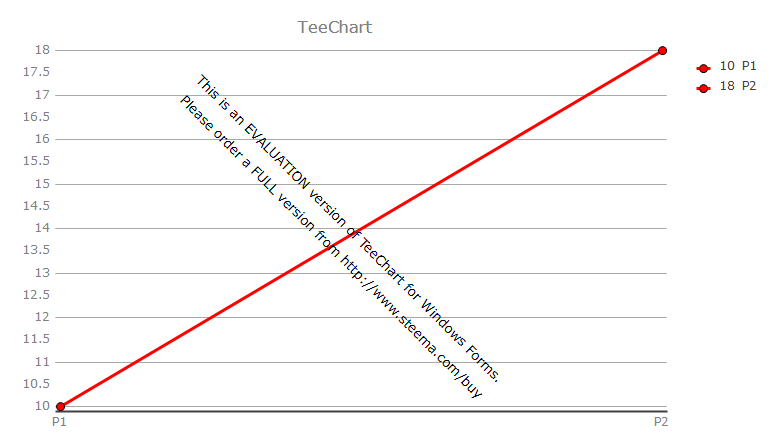
- Average.png (21.8 KiB) Viewed 52014 times
Code: Select all
public class ProductInfo
{
public string Product { get; set; }
public int Y { get; set; }
}
List<ProductInfo> rawList = new List<ProductInfo>()
{
new ProductInfo() { Product = "P1", Y = 10 },
new ProductInfo() { Product = "P2", Y = 18 }
};
public RawDataAverage()
{
InitializeComponent();
Line line = new Line(tChart1.Chart);
InitializeChart(rawList, line);
}
private void InitializeChart(List<ProductInfo> list, Line line)
{
line.LinePen.Width = 3;
line.Color = Color.Red;
line.Pointer.Visible = true;
line.Pointer.Style = PointerStyles.Circle;
line.YValues.DataMember = "Y";
line.LabelMember = "Product";
System.Console.WriteLine(rawList);
line.Add(rawList as IList);
}
RawData chart code as follow
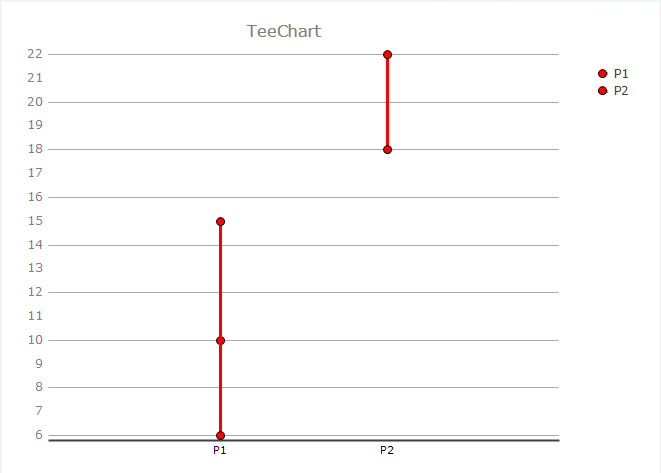
- RawData .png (9.87 KiB) Viewed 52014 times
Code: Select all
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
var line1 = new Line(tChart1.Chart);
var line2 = new Line(tChart1.Chart);
var product1 = "P1";
var product2 = "P2";
InitializeChart(line1, product1);
InitializeChart(line2, product2);
tChart1.Axes.Bottom.SetMinMax(-1, 2);
//with more than one series in the chart we need to set the
//labels manually like this:
tChart1.Axes.Bottom.Labels.Items.Add(0, product1);
tChart1.Axes.Bottom.Labels.Items.Add(1, product2);
tChart1.DoubleClick += new EventHandler(this.chart_DoubleClick);
}
public class ProductInfo
{
public string Product { get; set; }
public int Y { get; set; }
public int X { get; set; }
}
List<ProductInfo> list = new List<ProductInfo>()
{
new ProductInfo() { X = 0, Product = "P1", Y = 10 },
new ProductInfo() { X = 0, Product = "P1", Y = 15 },
new ProductInfo() { X = 0, Product = "P1", Y = 6 },
new ProductInfo() { X = 1, Product = "P2", Y = 22 },
new ProductInfo() { X = 1, Product = "P2", Y = 18 }
};
private void InitializeChart(Line line, string select)
{
line.LinePen.Width = 3;
line.Color = Color.Red;
line.Pointer.Visible = true;
line.Pointer.Style = PointerStyles.Circle;
line.YValues.DataMember = "Y";
line.XValues.DataMember = "X";
line.LabelMember = "Product";
line.Title = select;
line.Add(list.Where(x => x.Product == select).ToList() as IList);
}
}
Question1: When RawData Chart has a lot of data, the X-axis labels are stacked together. Want to clearly show how to deal with them?
The following figure shows an example of X-axis labels stacked together

- x label.png (13.42 KiB) Viewed 52014 times
Question2:Average Chart and RawData ,the X-axis is the same and I want to share an X-axis